User not logged in
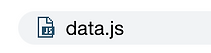
THE backend CODE
data.js File
// Programmer: Nabeel
import wixData from 'wix-data';
export function searchForDuplicateUsernames(value) {
return wixData.query("profile")
.eq("username", value.username)
.find()
.then((results) => {
return results.items.length;
})
.catch((err) => {
let errorMsg = err;
})
}
// emailAddress
export function searchForUsernamesIds(value) {
//This will check if the username and email is the same i.e, The username is not changed by the user
return wixData.query("profile")
.eq("username", value.username)
.eq("emailAddress" , value.emailAddress)
.find()
.then((results) => {
return results.items.length;
})
.catch((err) => {
let errorMsg = err;
})
}
export function profile_beforeInsert(item, context) {
return searchForDuplicateUsernames(item, context).then((res) => {
if(res > 0) {
return Promise.reject('This username is already taken');
}
return item;
});
}
export function profile_beforeUpdate(item, context, value) {
//courtesy of Salman from the Totally Codable Code community that helped finish this code
//fb.com/salman2301 or salmanhammed77@gmail.com preferred facebook
return searchForUsernamesIds(item, context).then((res) => {
if(res > 0) {
// the user name is not change but some other value is changed
return Promise.resolve('Updated the changes');
} else {
// The username is changed , Checking if the username value is taken by some other member or not
return searchForDuplicateUsernames(item, context).then((res) => {
if(res > 0){
return Promise.reject('This username is already taken');
}
})
}
return item;
});
}
export function onUpDate(value) {
return wixData.query("profile")
.eq("username", value.username)
.find()
.then((results) => {
return results.items.length;
})
.catch((err) => {
let errorMsg = err;
})
}